Fetch vs. Axios in JavaScript: Story of Two HTTP Clients 📡
- Abhishek Kumar
- 28 Aug, 2024
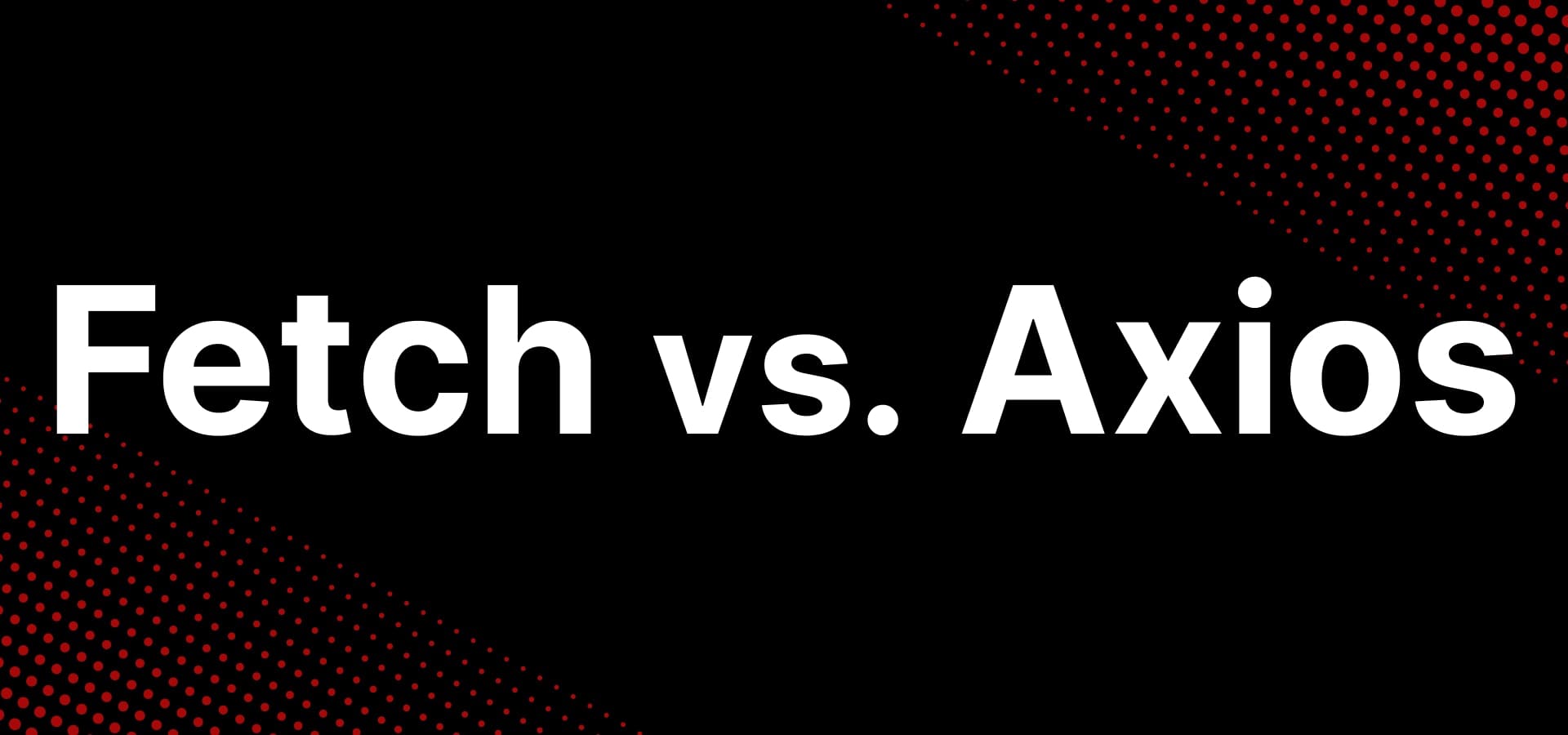
When it comes to making HTTP requests in JavaScript, two names often pop up: Axios and Fetch. If you’re a developer with a couple of years under your belt—or maybe even a fresh-faced newbie—you’ve probably heard of them. But what’s the big deal? Why are there two ways to do the same thing? Let’s dive into the differences and see why one might be your new best friend while the other might just be… a good acquaintance. 😉
The Basics: What Are They?
-
Fetch is a built-in JavaScript function that’s been around since ES6 (2015). It allows you to make HTTP requests to servers—like GET, POST, PUT, DELETE, and so on.
-
Axios is a promise-based HTTP client that was originally built for Node.js and has since become a popular choice in the frontend world. It’s basically Fetch on steroids, with more features packed in.
Syntax: Plain and Simple vs. Feature-Packed
-
Fetch Syntax:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Fetch is simple and straightforward but can get a bit cumbersome when dealing with more complex scenarios, like handling errors or managing multiple requests.
-
Axios Syntax:
axios.get('https://api.example.com/data') .then(response => console.log(response.data)) .catch(error => console.error('Error:', error));
Axios offers a cleaner syntax, especially when handling larger applications or more complex request configurations. Notice how the response data is accessed directly—no need to manually parse the JSON.
Error Handling: One is a Bit of a Drama Queen
-
Fetch: By default, Fetch only rejects a promise if there’s a network failure. If the server responds with a 404 or 500 error, Fetch considers the request successful unless you manually check the
response.ok
property.fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
-
Axios: Axios automatically rejects the promise for HTTP status codes that indicate an error (like 404 or 500). It’s built-in error handling for when things go wrong. 🎭
axios.get('https://api.example.com/data') .then(response => console.log(response.data)) .catch(error => console.error('Error:', error));
Interceptors: Axios Flexes Its Muscles
-
Fetch: Doesn’t have built-in support for request/response interceptors. You’d have to manually write middleware-like code to achieve this.
-
Axios: One of Axios’s superpowers is its ability to add interceptors. These allow you to run your code or modify requests/responses before they are handled by
.then()
or.catch()
. Great for adding things like authentication tokens to requests automatically.axios.interceptors.request.use(config => { config.headers.Authorization = `Bearer ${token}`; return config; });
File Transfer: Fetching and Uploading files
When you’re dealing transferring files over HTTP, both Axios and Fetch have their way of handling the job. especially when it comes to tracking how your files are transferring. Let’s break it down in a way that’s easy to digest, even if you’re relatively new to this.
-
Axios: Axios makes file transfers easy with its
onDownloadProgress
andonUploadProgress
options. These features let you see how your file transfer is going in real time.onDownloadProgress
: This lets you track how much of a file has been downloaded. You get updates on the number of bytes downloaded and the total size, which is great for showing a progress bar or celebrating each time data arrives.onUploadProgress
: This tracks how much of a file has been uploaded. You get updates on the upload progress, making it easier to inform users about how their upload is going and reducing the boredom of long waits.axios.get('/some-url-of-large-file', { onDownloadProgress: (progressEvent) => { const percentCompleted = Math.round((progressEvent.loaded * 100) / progressEvent.total); console.log(`Download Progress: ${percentCompleted}%`); }, responseType: 'blob' // or 'arraybuffer' if you need raw data })
-
Fetch: Fetch, on the other hand, doesn’t come with built-in support for tracking download or upload progress. It’s a bit like having a camera without a viewfinder—you can still use it, but you don’t get as much immediate feedback.
While Fetch doesn’t natively track download progress, you can achieve this using streams
fetch('/some-url-of-large-file') .then(response => { const total = response.headers.get('Content-Length'); let loaded = 0; response.body.getReader().read().then(function processText({ done, value }) { if (done) return; loaded += value.length; const percentCompleted = Math.round((loaded * 100) / total); console.log(`Download Progress: ${percentCompleted}%`); return response.body.getReader().read().then(processText); }); });
Support for Older Browsers
- Fetch: Requires a polyfill for older browsers like Internet Explorer. It’s a modern API, so it’s not natively supported everywhere.
- Axios: Works with a broader range of browsers out of the box. If you’re building something that needs to support legacy systems, Axios might save you some headaches.
Fetch is like bringing a fresh, modern gadget to a party—cool, but you might need an adapter (or polyfill) for it to work with the older tech. Axios? It’s got that universal charger ready to go. 🔌
To Fetch or Not to Fetch?
If you need a simple, straightforward way to make HTTP requests and don’t mind a bit of extra work handling errors or configurations, Fetch will do just fine. But if you’re building something more complex, or just want a smoother ride with extra features like interceptors, Axios is the way to go.
In the end, both tools will get you where you need to go. It’s just a matter of whether you want to pedal your way there or take the easy road with some extra horsepower.
Happy coding! 👨🏼💻
If you like this article, don't forget to share it with your friends and colleagues.